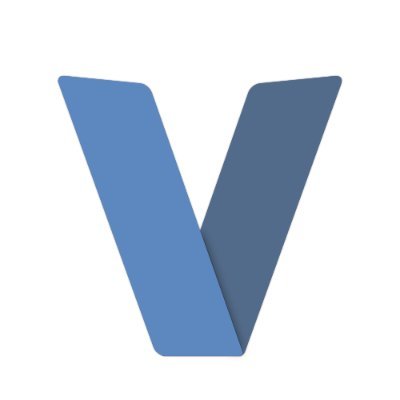
3/3: Arena allocation and manual memory management are also available, so V gives the developer full control.
42 Likes
On the benefits of using C as a language backend
V uses C as the primary backend.
Many people find this choice strange, and one of the most common comments/suggestions is "Why not use LLVM?"
...